FlipDotDisplay
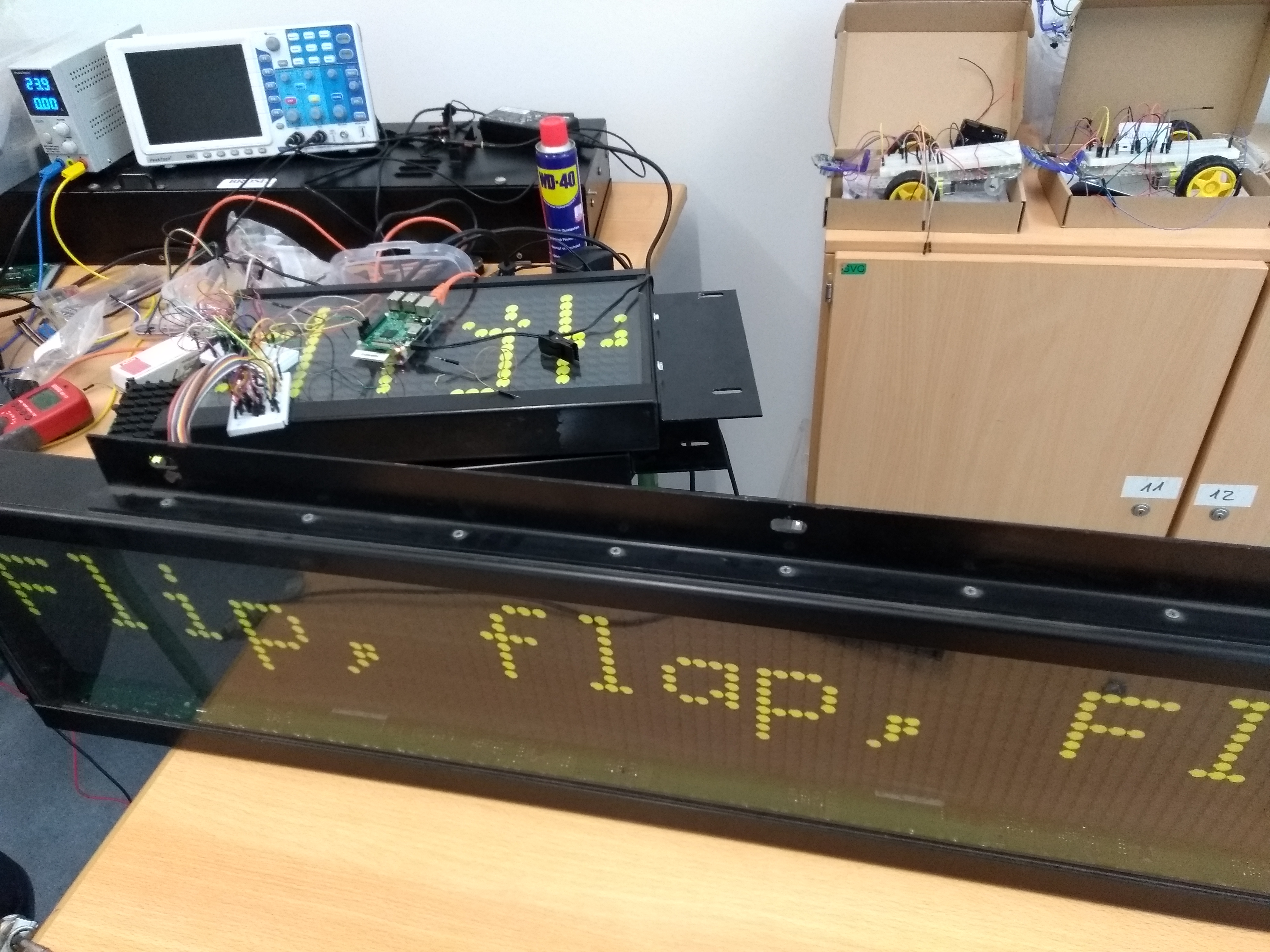
Module fffserial
The serial module relies on an ardiuno connected to the display that runs
the firmware
:
+----------+ +---------+ +-----------------+
|Raspberry |--[serial]----| Arduino |-----| flipdot-display |
|Pi | | | | |
+----------+ +---------+ +-----------------+
Module for communicating with the display using a serial interface. The display is connected to an arduino. This packages helps during the communication with the device over a serial interface.
- class fffserial.SerialDisplay(width=4, height=3, serial_device='/dev/ttyUSB0', baud=9600, buffered=True)[source]
Serial Display sending commands to an arduino connected to the display. Each command starts with a byte with a command identifier. The following bytes are the command parameters.
- DIMENSION = 144
The following two bytes are the width and height of the display.
- ECHO = 240
The following byte is returned.
- LED_BRIGTHNESS = 132
Set the brightness of the LED. The following byte is the brightness.
- PICTURE = 129
The following bytes are the picture data (row by row).
- PXRESET = 130
Removing a pixel. The following two Bytes X, Y with information about the pixel to reset.
- PXSET = 131
The following two Bytes X, Y with information about the pixel to set.
- __init__(width=4, height=3, serial_device='/dev/ttyUSB0', baud=9600, buffered=True)[source]
Create serial display with given dimension. If buffered is True, all calls to px() will write into an internal buffer until a call to show() will send the data.
- __module__ = 'fffserial'
Module flipdotdisplay
The module must be connected to a RaspberryPi which in turn is connected to a port expander. The port expander itself is controlling the flipdot display. Each display is segmentd into modules. Each of these modules must be connected seperately to one GPIO port on the Raspberry Pi.
+----------+ +---------+ +-----------------+
|Raspberry |--[I²C SDA]---| Port- |-----| flipdot-display |
|Pi | | epander | |_________________|
| |--[I²C SCL]---| |-----|mod1|mod2|mod3 |
+----------+ +---------+ +-----------------+
||| | | |
+++---------[one wire per module]--------+----+-----+
ATTENTION: The following implementation of a flipdotdisplay was the first attempt that used a portexpander to control the display. It was replaced by a arduino based solution that is more reliable and faster. The code is still here for reference but is no longer supported.
The flipdotdisplay package allows for controlling a physical flipdotdisplay. It relies on a portexpander that is connected to the display via I²C or SPI on one hand and to a RaspberryPi on the other hand.
Suppose we would like to show the following pattern in the top left corner of the display:
o.
.o
First a FlipDotDisplay
display must be created. We use the default
parameters here.
>>> import flipdotdisplay
>>> fdd = flipdotdisplay.FlipDotDisplay()
The new display can now be used to set the pixels
with the px()
method.
>>> fdd.px(0,0, True)
>>> fdd.px(0,1, False)
>>> fdd.px(1,0, False)
>>> fdd.px(1,1 True)
After setting the pixels we need one final step to make them visible on the
display with show()
.
>>> fdd.show()
A list of default GPIO pins for the modules is 14, 15, 18, 23, 24.
- class flipdotdisplay.FlipDotDisplay(address=32, width=28, height=13, module=[18])[source]
- __annotations__ = {}
- __init__(address=32, width=28, height=13, module=[18])[source]
Create a display connected via a port expander on the given I²C-address. The given module list contains GPIO-ports that connect the RaspberryPi with the the module in the display.
- __module__ = 'flipdotdisplay'
Module util
This module contains helper methods for the handling of flipdotdisplays.
- util.draw_surface_on_fdd(surface: pygame.Surface, flipdotdisplay)[source]
Draw the surface onto the display. You need to invoke show() afterwards to make it visible. Black pixels (rgb 0,0,0) are considered black and turned off, other colors are considered pixels turned on. The clipping area of the surface can be modified to draw only part of the surface. A demo application is available in demos.PygameSurfaceDemo.
- util.draw_text_on_fdd(text, fontname, fontsize, flipdotdisplay)[source]
Draw text on a display.
>>> import net >>> import util >>> fdd = net.RemoteDisplay("taylorpi.local") Remote display will send data to taylorpi.local on port 10101 >>> util.draw_text_on_fdd("Hallo", "Arial", 9, fdd) >>> fdd.show()
Text On The Display
The class flipdotfont.TextScroller
can be used to write text onto the display.
Another way to bring text onto the dispoay is by using util.draw_text_on_fdd()
.
- class flipdotfont.Font(filename, width, height)[source]
read a .bdf font file letter() gets a character and returns a list of 8-bit-integers.
example 3x4 “T”
- [0b11110000,
0b01100000, 0b01100000]
- class flipdotfont.TextScroller(flipdotdisplay, text, font)[source]
Write Text on Flipdotdisplays. A simple usage with a FlipDot-Simulator is shown in the following.
>>> import flipdotfont >>> import flipdotsim >>> import time >>> fds = flipdotsim.FlipDotSim(28, 13) >>> t = flipdotfont.TextScroller(fds, "Hello world.", ... flipdotfont.big_font()) >>> for _ in range(20): ... t.scrolltext() ... fds.show() ... time.sleep(0.1)